This guide will show how to test a Python Flask application using Humlix.
Let's start by creating a simple example with only one endpoint that returns a given car's index in a database.
Can you discover the bug in the code below?
class Car:
def __init__(self):
self.cars = ["Ford", "Volvo", "BMW"] #Fake db
def getCarIndex(self, user):
if (user['hasCar'] == True):
return self.cars.index(user['car'])
else:
return 0
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/cars', methods=['POST'])
def cars():
json = request.json
car = Car()
carIndex = car.getCarIndex(json)
return jsonify(carIndex = carIndex)
The JSON data passed to the endpoint is structured like this:
{
"id" : 1,
"username" : "humlix",
"hasCar" : true,
"car" : "Volvo"
}
To test this application, we would typically write traditional unit tests and could easily end up missing edge cases causing problems.
To complement our unit tests, we test the functionality with Humlix as well. In the picture below, Humlix shows that it only took four tests to get a 500 Internal Server Error.
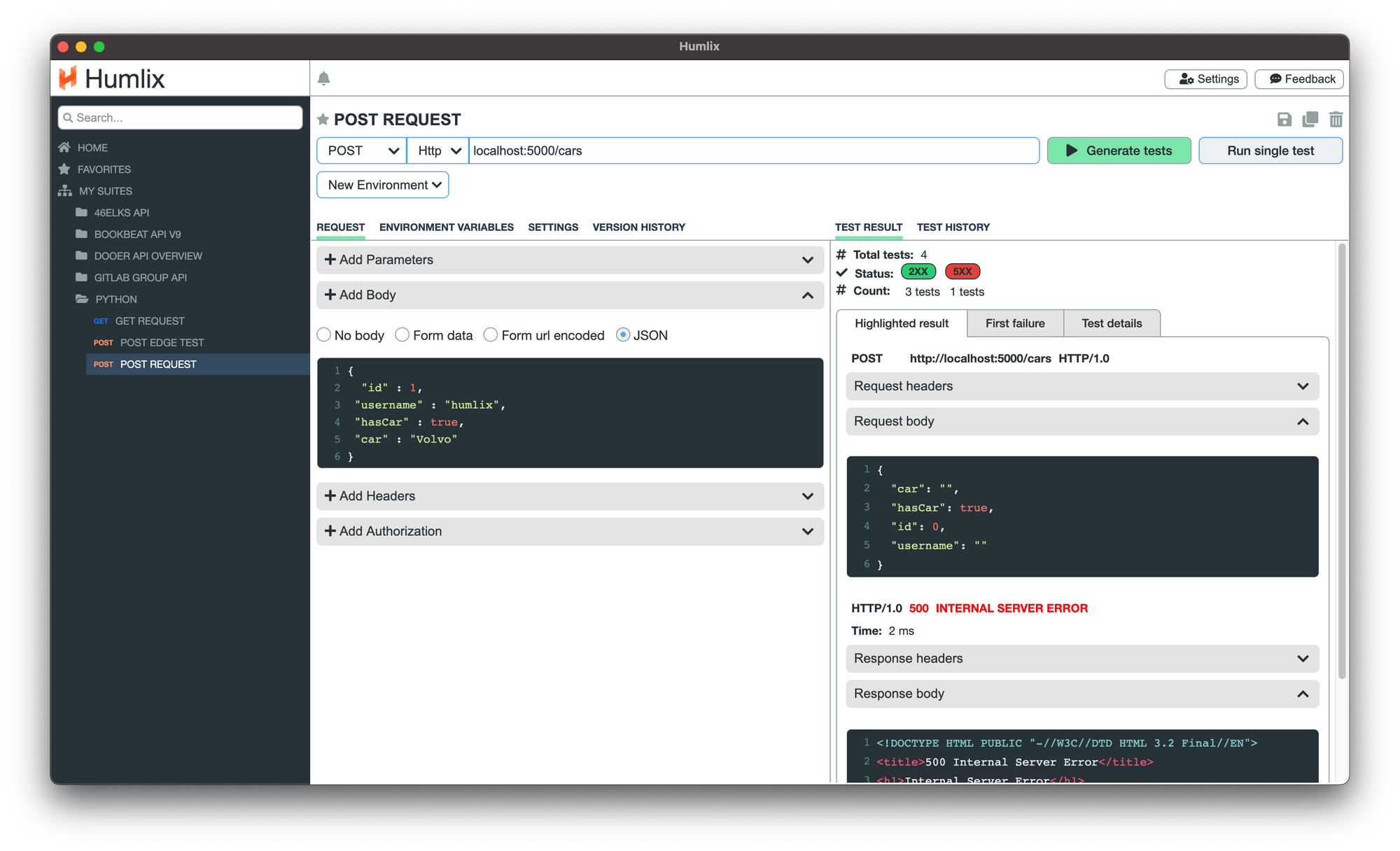
Zooming in on the details, seeing the smallest test data needed to repeat the problem is possible.
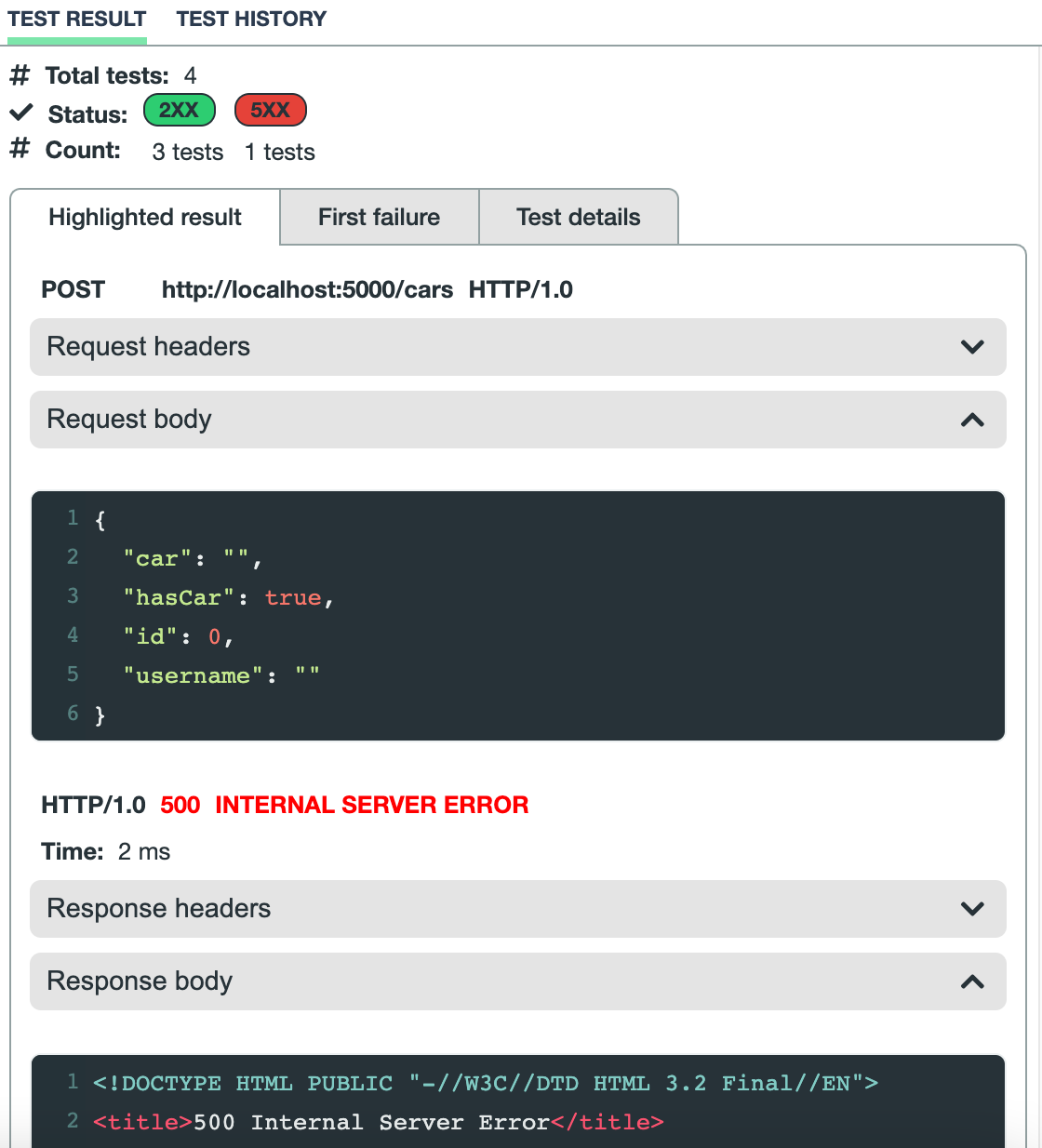
Looking at the log, it becomes evident that we are indexing into an array, but the value doesn't exist. Therefore we need to fix Line 7 in the code return self.cars.index(user['car']) to handle the test case Humlix created above.
[2020-12-06 13:38:52,493] ERROR in app: Exception on /cars [POST]
Traceback (most recent call last):
File "/Users/env/flaskexample/venv/lib/python3.9/site-packages/flask/app.py", line 2447, in wsgi_app
response = self.full_dispatch_request()
File "/Users/env/flaskexample/venv/lib/python3.9/site-packages/flask/app.py", line 1952, in full_dispatch_request
rv = self.handle_user_exception(e)
File "/Users/env/python/flaskexample/venv/lib/python3.9/site-packages/flask/app.py", line 1821, in handle_user_exception
reraise(exc_type, exc_value, tb)
File "/Users/env/flaskexample/venv/lib/python3.9/site-packages/flask/_compat.py", line 39, in reraise
raise value
File "/Users/env/flaskexample/venv/lib/python3.9/site-packages/flask/app.py", line 1950, in full_dispatch_request
rv = self.dispatch_request()
File "/Users/env/flaskexample/venv/lib/python3.9/site-packages/flask/app.py", line 1936, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "/Users/env/flaskexample/hello.py", line 35, in cars
carIndex = car.getCarIndex(json)
File "/Users/env/flaskexample/hello.py", line 7, in getCarIndex
return self.cars.index(user['car'])
ValueError: '' is not in list
127.0.0.1 - - [06/Dec/2020 13:38:52] "POST /cars HTTP/1.1" 500 -
That was a quick walkthrough on using Humlix to discover bugs caused by edge cases that are hard to find otherwise.
Download Humlix and try for yourself.